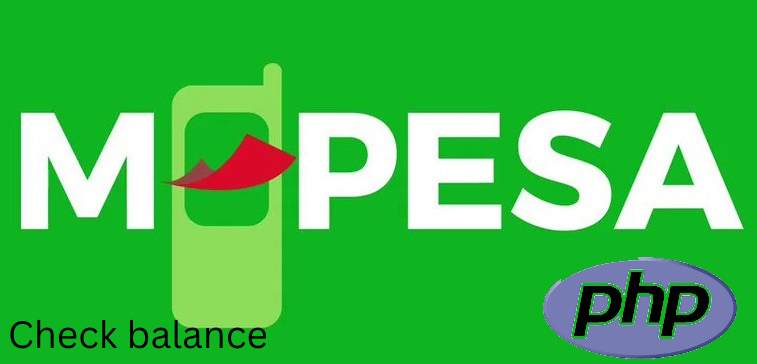
How To Intergrate M-Pesa Check Balance With Your Website Php
In this tutorial Ill show you how to intergrate m-pesa check balance with your website in php
1.Create a check balance file and paste in the following code:
<?php
/* access token */
$consumerKey = ‘YgreYNuYW5xIVooFrZgIhQMvvBGr2Pe2’; //Fill with your app Consumer Key
$consumerSecret = ‘699oGg0C1XdMoWOA’; // Fill with your app Secret
$headers = [‘Content-Type:application/json; charset=utf8’];
$access_token_url = ‘https://sandbox.safaricom.co.ke/oauth/v1/generate?grant_type=client_credentials’;
$curl = curl_init($access_token_url);
curl_setopt($curl, CURLOPT_HTTPHEADER, $headers);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt($curl, CURLOPT_HEADER, FALSE);
curl_setopt($curl, CURLOPT_USERPWD, $consumerKey.’:’.$consumerSecret);
$result = curl_exec($curl);
$status = curl_getinfo($curl, CURLINFO_HTTP_CODE);
$result = json_decode($result);
$access_token = $result->access_token;
curl_close($curl);
/* main request */
$bal_url = ‘https://sandbox.safaricom.co.ke/mpesa/accountbalance/v1/query’;
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $bal_url);
curl_setopt($curl, CURLOPT_HTTPHEADER, array(‘Content-Type:application/json’,’Authorization:Bearer ‘.$access_token)); //setting custom header
$curl_post_data = array(
//Fill in the request parameters with valid values
‘Initiator’ => ‘testapi0288’, # initiator name -> For test, use Initiator name(Shortcode 1)
‘SecurityCredential’ => ‘hDTg2NI3tiAmaDSuoiJc1AdLTui45L789y2/ZUCdgGUg9S5udY5gYdXVhYC62J4gNpgm2CUcIcL0BTRCDlOMPVbygZNrGXO2fXH9+XxxLCWDO/3VxPaeYO2e6a3CZ1rBK95nTputPmPSCgr8dTTlc7hWz0kN8tLDaydFpA6RnoPLEhIq96QEt8l/HXUc9yzd5SvOmJ+Ww+8lF1ufM4ip0M2Cz5g/VXCPpwx8SahjU10Ems2V8A6yzxfT7f3izHF6wxZ2O9E1Rup6R2HJLkyp5EjHaS7cTvFlmp2t9Vw9jL9n8/uZsFTQ8jS3bY9cHbDPijozb0hb9JpfDKSvuoGlUA==’, #Base64 encoded string of the Security Credential, which is encrypted using M-Pesa public key
‘CommandID’ => ‘AccountBalance’, # Command ID, Possible value AccountBalance
‘PartyA’ => ‘600288’, # ShortCode 1, or your Paybill(During Production)
‘IdentifierType’ => ‘4’,
‘Remarks’ => ‘Bal’, # Comments- Anything can go here
‘QueueTimeOutURL’ => ‘https://mpesa.uxtcloud.com/mapi/balance/bal_callback_url.php’, # URL where Timeout Response will be sent to
‘ResultURL’ => ‘https://mpesa.uxtcloud.com/mapi/balance/bal_callback_url.php’ # URL where Result Response will be sent to
);
$data_string = json_encode($curl_post_data);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, $data_string);
$curl_response = curl_exec($curl);
print_r($curl_response);
echo $curl_response;
?>
2.Create a callback file and paste in the below code:
<?php
$callbackResponse = file_get_contents(‘php://input’);
$logFile = “bal-callback-response.json”;
$log = fopen($logFile, “a”);
fwrite($log, $callbackResponse);
fclose($log);