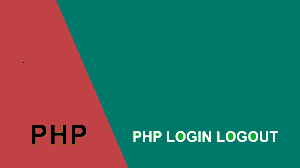
How To Create Login Signup In Php Mysqli
In your project folder create another folder and name it config
In config folder create a file dbconnect.php and paste in the following code:
<?php
$host = 'localhost';
$user = 'root';
$pass = '';
$db = '';
$con = mysqli_connect($host,$user,$pass,$db) or die('Unable to Connect');
?>
In your project folder create index.php file and paste in the following code:
<?php
include_once('config/dbconnect.php');
if(empty($_SESSION['username'])){
header('Location:login.php');
}
?>
<!DOCtype="html">
<html>
<head>
<title>Signup</title>
</head>
<body>
<div>
<h2>Logged in,Username <?php echo $_SESSION['username']; ?></h2>
<p><a href="logout.php">Log out</a></p>
</div>
</body>
</html>
Create a login.php file and paste in the following code:
<?php
include_once('config/dbconnect.php');
?>
<!DOCtype="html">
<html>
<head>
<title>Login</title>
</head>
<body>
<div>
<form method="POST" action="">
<label>Username/Email</label><input type="text" name="username"/><br/>
<label>Password</label><input type="password" name="password"/><br/>
<input type="submit" name="login" value="Login"/>
</form>
</div>
</body>
</html>
<?php
if(isset($_POST['login'])){
$username = $_POST['username'];
$password = $_POST['password'];
$password = md5($password);
$sql = "SELECT * FROM users WHERE (username = '$username' OR email = '$username') AND password = '$password'";
$query = mysqli_query($con,$sql);
$rows = mysqli_fetch_row($query);
if($rows > 0){
session_start();
$_SESSION['username'] = $rows['username'];
$_SESSION['email'] = $rows['email'];
}else{
header('Location:login.php?error=1');
}
}
?>
Create a signup.php file and paste in the following code:
<?php
include_once('config/dbconnect.php');
?>
<!DOCtype="html">
<html>
<head>
<title>Signup</title>
</head>
<body>
<div>
<form method="POST" action="">
<label>Username</label><input type="text" name="username"/><br/>
<label>Email</label><input type="email" name="email"/><br/>
<label>Password</label><input type="password" name="password"/><br/>
<input type="signup" name="signup" value="Signup"/>
</form>
</div>
</body>
</html>
<?php
if(isset($_POST['signup'])){
$username = $_POST['username'];
$email = $_POST['email'];
$password = $_POST['password'];
$password = md5($password);
$sql = "INSERT INTO users (username,email,password) VALUE '$username','$email','$password'";
if(mysqli_query($con,$sql)){
header('Location:login.php');
}
}
?>
Create a logout.php file and paste in the following code:
<?php
include_once('config/dbconnect.php');
session_destroy();
header('Location:login.php');
?>