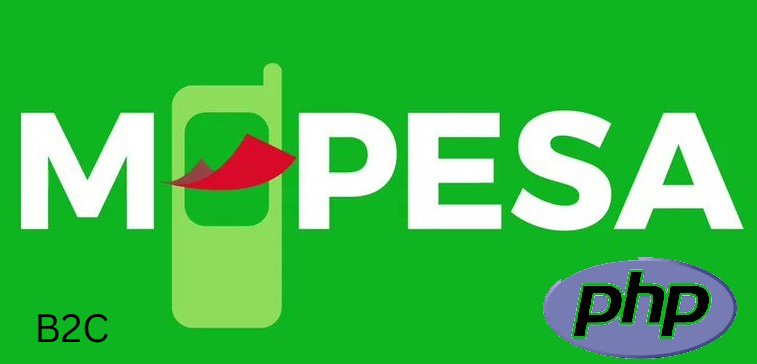
How To Intergrate M-Pesa B2C With Your Website In Php
In this tutorial Ill show you how to intergrate m-pesa b2c with your website in php
1.Create a b2c file and paste in the following code:
<?php
/* Urls */
$access_token_url = ‘https://sandbox.safaricom.co.ke/oauth/v1/generate?grant_type=client_credentials’;
$b2c_url = ‘https://sandbox.safaricom.co.ke/mpesa/b2c/v1/paymentrequest’;
/* Required Variables */
$consumerKey = ‘YgreYNuYW5xIVooFrZgIhQMvvBGr2Pe2’; # Fill with your app Consumer Key
$consumerSecret = ‘699oGg0C1XdMoWOA’; # Fill with your app Secret
$headers = [‘Content-Type:application/json; charset=utf8’];
/* from the test credentials provided on you developers account */
$InitiatorName = ‘apitest361’; # Initiator
$SecurityCredential = ‘RHHsFx9loY3TtMm7RYJA91YIT9C1wFIULPyD2m+chN27Pnj+NyA2nQHguT4C3ESySnBiuvs3iyQW96eH9x0sggTvDnx/gBu9ro3YKFFBMoZ7YyVVFGJo2QbP3pn5FNz9lGyEhfdS+GzXTT/xprYUcscnxuRKbtlEhh6Ebq/kzGOoCwZK3pguJaCOgGAR9O/QqlNqPmUtG382Mm/DUC8wR1qF/CzuqRCrXKlH1GaZIi7kjBVVTFBsAAs4FyGEuMMMiE3FZQGSxjoTGAS53T/aEKCWcHFZvon0SWJpKyy1kWcfEpdIrdquSwh937dYBrWoFF//IG8uUb7Hoi6eZ9mLww==’;
$CommandID = ‘SalaryPayment’; # choose between SalaryPayment, BusinessPayment, PromotionPayment
$Amount = ’10’;
$PartyA = ‘600288’; # shortcode 1
$PartyB = ‘254708374149’; # Shortcode 2
$Remarks = ‘Salary’; # Remarks ** can not be empty
$QueueTimeOutURL = ‘https://mpesa.uxtcloud.com/mapi/b2c/B2CResultURL.php’; # your QueueTimeOutURL
$ResultURL = ‘https://mpesa.uxtcloud.com/mapi/b2c/B2CResultURL.php’; # your ResultURL
$Occasion = ‘Salary march’; # Occasion
/* Obtain Access Token */
$curl = curl_init($access_token_url);
curl_setopt($curl, CURLOPT_HTTPHEADER, $headers);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt($curl, CURLOPT_HEADER, FALSE);
curl_setopt($curl, CURLOPT_USERPWD, $consumerKey.’:’.$consumerSecret);
$result = curl_exec($curl);
$status = curl_getinfo($curl, CURLINFO_HTTP_CODE);
$result = json_decode($result);
$access_token = $result->access_token;
curl_close($curl);
/* Main B2C Request to the API */
$b2cHeader = [‘Content-Type:application/json’,’Authorization:Bearer ‘.$access_token];
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $b2c_url);
curl_setopt($curl, CURLOPT_HTTPHEADER, $b2cHeader); //setting custom header
$curl_post_data = array(
//Fill in the request parameters with valid values
‘InitiatorName’ => $InitiatorName,
‘SecurityCredential’ => $SecurityCredential,
‘CommandID’ => $CommandID,
‘Amount’ => $Amount,
‘PartyA’ => $PartyA,
‘PartyB’ => $PartyB,
‘Remarks’ => $Remarks,
‘QueueTimeOutURL’ => $QueueTimeOutURL,
‘ResultURL’ => $ResultURL,
‘Occasion’ => $Occasion
);
$data_string = json_encode($curl_post_data);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, $data_string);
$curl_response = curl_exec($curl);
print_r($curl_response);
echo $curl_response;
?>
2.Create a callback file and paste in the below code:
<?php
$callbackResponse = file_get_contents(‘php://input’);
$logFile = “b2c-callback-response.json”;
$log = fopen($logFile, “a”);
fwrite($log, $callbackResponse);
fclose($log);